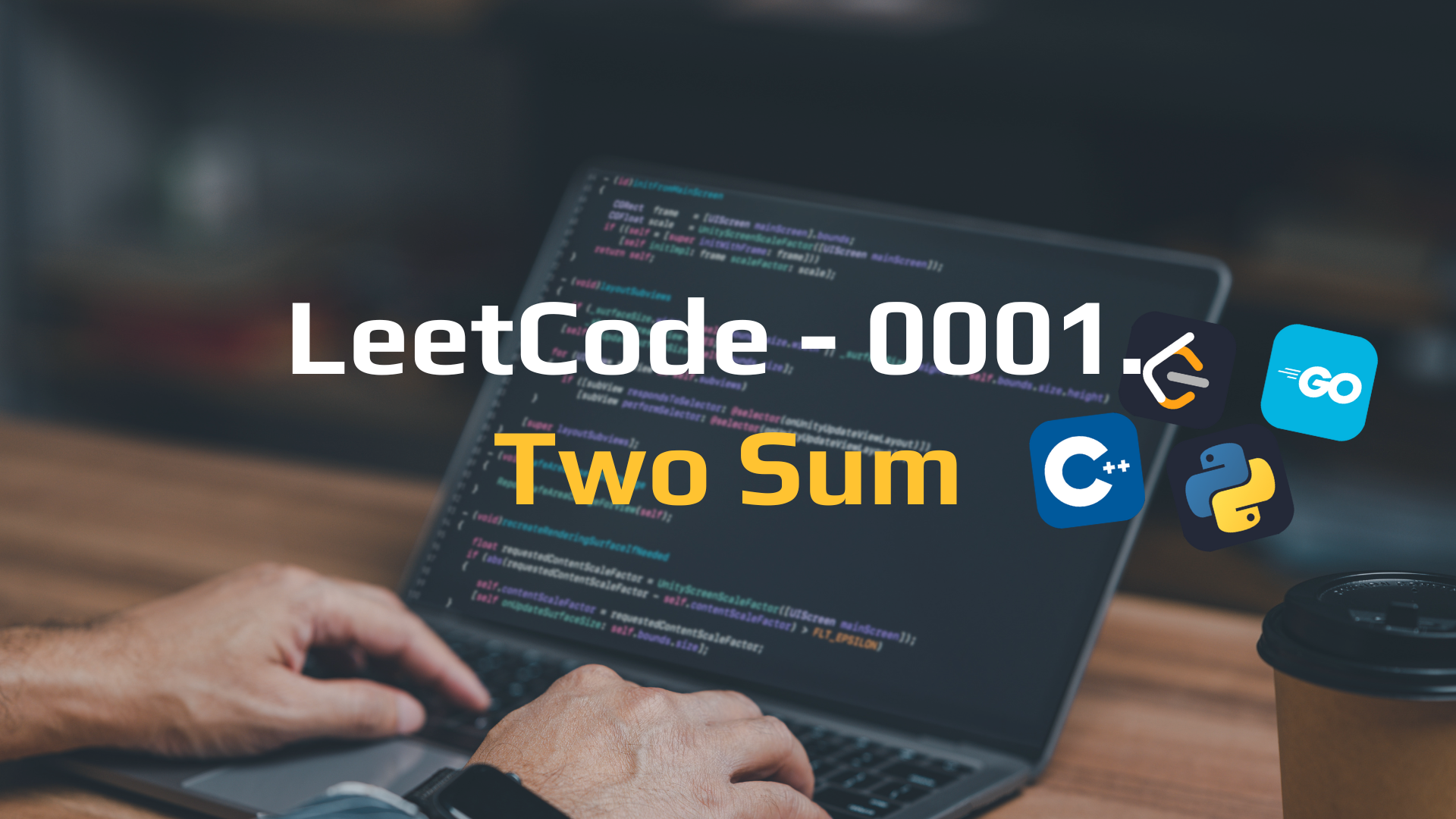
LeetCode 0001. Two Sum - Hash Map Solution | Go, Python, C++
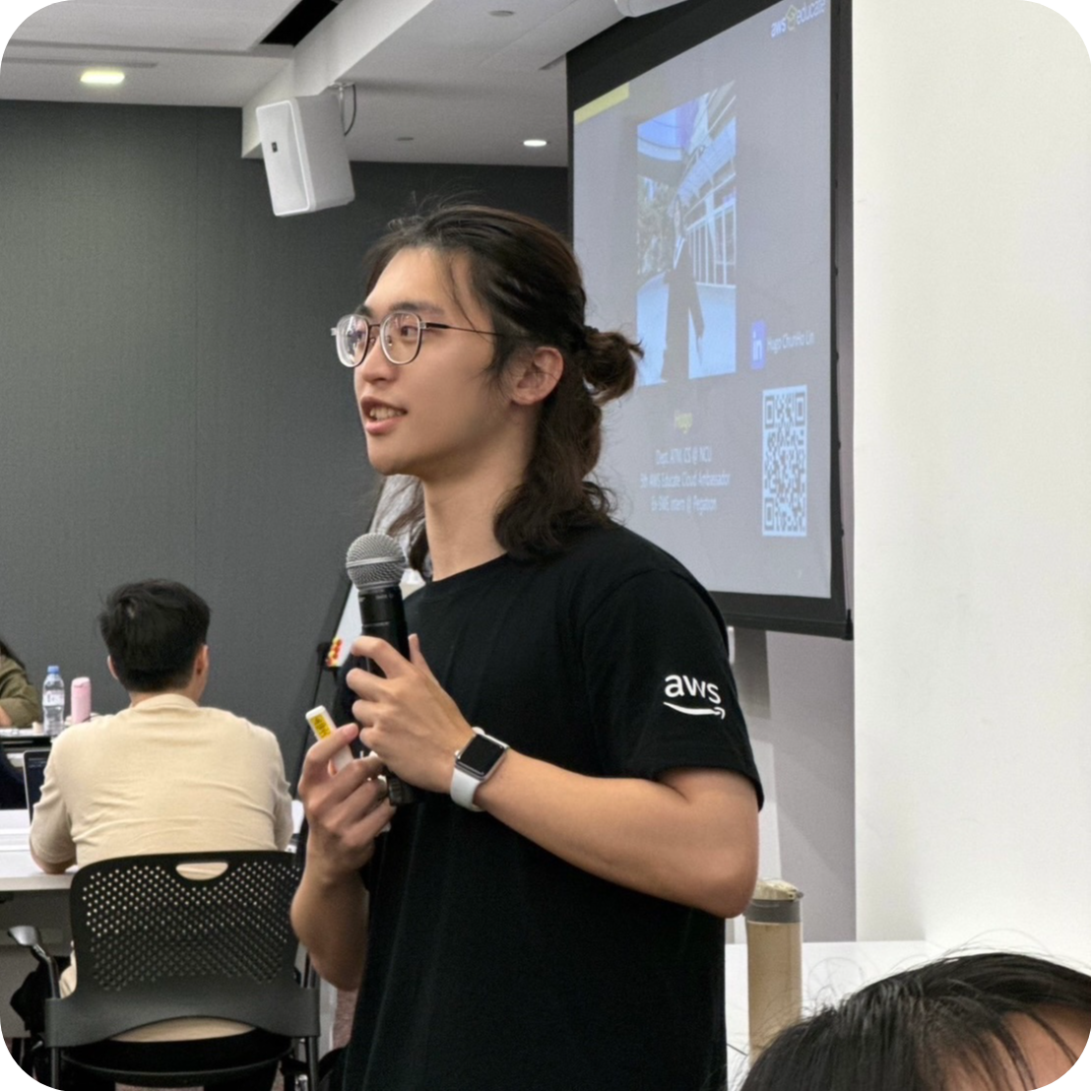
Link ππ» 1. Two Sum
Description
Given an array of integers nums
and an integer target
, return indices of the two numbers such that they add up to target
.
You may assume that each input would have exactly one solution, and you may not use the same element twice.
You can return the answer in any order.
Example 1:
- Input:
nums = [2,7,11,15], target = 9
- Output:
[0,1]
- Explanation:
Because nums[0] + nums[1] == 9, we return [0, 1].
Example 2:
- Input:
nums = [3,2,4], target = 6
- Output:
[1,2]
Example 3:
- Input:
nums = [3,3], target = 6
- Output:
[0,1]
Constraints:
2 <= nums.length <= 104
-109 <= nums[i] <= 109
-109 <= target <= 109
Only one valid answer exists.
Follow-up: Can you come up with an algorithm that is less than O(n2)
time complexity?
Intuition
Use HashMap to keep numbers and their indices we found.
Note
Create a Hash Table in GO
with make
function Golang Maps , and use map[key] = value
to set the value of the key.
1 | numMap := make(map[int]int) |
Note
How to Work with maps? Go maps in action#Working with maps
A two-value assignment tests for the existence of a key:
1 | i, ok := m["route"] |
In this statement, the first value (i)
is assigned the value stored under the key βrouteβ. If that key doesnβt exist, i is the value typeβs zero value (0)
. The second value (ok)
is a bool
that is true if the key exists in the map, and false if not.
To test for a key without retrieving the value, use an underscore in place of the first value:
1 | _, ok := m["route"] |
To iterate over the contents of a map, use the range keyword:
1 | for key, value := range m { |
Approach
- Traverse the
nums
array and store the difference between thetarget
and the currentnumber
as thekey
and theindex
as thevalue
in the HashMap. - If the current
number
is already in the HashMap, return theindex
of the currentnumber
and theindex
stored in the HashMap. - We still need to return an empty array if there is no solution.
Complexity
Time complexity:
Space complexity:
Code
1 | func twoSum(nums []int, target int) []int { |
1 | class Solution: |
1 | class Solution { |